Introduction: Binary Code to ASCII Letters Teaching Game
When I first learned about computers in college (1963) I never quite understood the relationship between binary/octal/hex/ascii/op-codes and other details of computer innards. In the mid 80s I built an IMSAI 8080 and it had a set of switches to input data and I finally figured it out. That computer is long gone but the excitement of that learning experience has always stuck with me. Fast forward to 2020: I volunteer at a museum in Chatham, MA, and we desired some small STEM exhibits to teach basic math and engineering to school kids in the fourth grade and up. I built this game to engage kids and teach them how computers use binary numbers to encode alphabet letters. Here's the challenge question:
Question:
If computers only know about ones and zeros, how do computers make letters?
The answer is "there is a code", but kids get to have hands-on experience flipping the eight toggle switches to spell their names one letter at a time. They refer to the list of letters and their ASCII equivalent 8 digit binary code, flip the switches with satisfying clicks, and press the green Enter button to add on a letter. Make a mistake? Press the red Reset button to clear the display and start over. Youngsters will play the "game", show their friends how to do it, and do it over and over... 16 letters is enough for most names as well as funny stuff like poopoohead.
Supplies
8 toggle switches SPST
8 tricolor LEDs, common Anode (I used RGB, but RG would work)
8 2.2K resistors
2 SPST pushbuttons
16x2 LCD Panel
Arduino Mega or similar
10K potentiometer
1K potentiometer
9 volt power supply to power the Arduino which then feeds 5volts to LCD and LEDs
Step 1: Study the Inspiration for My Project
This project was inspired by a great Instructable from 2018 that showed how to use a 16 x 2 LCD Panel and an Arduino. Read that one before proceeding, because I'm a lousy typist and he gave us an extensive and excellent tutorial on the circuit basics you'll be employing. Here's a link to it, along with a big tip of the hat to that author, Indoorgeek. Thanks and well done!
https://www.instructables.com/LCD-Trainer-Kit/
Step 2: Gather the Parts and Drill a Panel
I made my first version on a cardboard box, as a proof of concept and layout. There is nothing special about the panel. It could have been wood or masonite, but I wanted something that kids' fingers wouldn't wear out and that could be wiped clean. My final version is a sandwich of a white plastic panel about 1/4" thick topped with matching 1/8" clear plastic. That way I could put paper sheets with text and lables in between the layers. The only tricky part was laying out the holes for the switches and the LEDs and cutting a square window to mount the LCD panel. Taping the two layers together before drilling assured that the holes would line up.
Step 3: Mount and Wire the Parts
Mounting the parts is straightforward. The switches and LEDs mount in holes. The LCD panel is hot glued in place. The Arduino is held on by sticky backed velcro.
The wiring and the potentiometers are just hanging in the air as the panel would be mounted onto a box that would keep inquisitive fingers away.
The wiring diagram is pretty much self explanatory. The only part that may require a bit of experimentation is the three-color LEDs. I use only the Red and Green colors. When any of the eight toggle switches are up, representing a 1 in binary the LED above it shows Green. When a switch is down representing a 0 in binary the LED above it shows Red.
The wiring diagram shows that when a toggle switch is open the green LED will be on, fed 5 volts via a 2.2K Resistor. When the switch is closed, the red LED is connected to ground, it illuminates, and that "LOW" signal is passed to the Arduino which interprets it as a zero (binary 0). I know this seems funny but this behavior of the LEDs means I didn't need double pole switches. It is possible that both red and green are on, but the red light is what we see.
Attachments
Step 4: Program the Arduino
The sketch (the program) is pretty simple. The .ino file can be downloaded, but here is a text version:
//For ASCII Exhibit , here’s a readable text version of Arduino file “ASCIISwitchesFn2Int.ino”
//Read 8 switche and display ascii equivalent character on the 16X2 LCD
//this version of the Arduino software adds
// asciiSwitches as a function and also the interrupt handler enterAscii:
#include "LiquidCrystal.h"
// initialize the library by providing the number of pins to connect to LCD
LiquidCrystal lcd(8, 9, 4, 5, 6, 7);
int n = 0; // character position in second line of display
bool enter; //boolean to test recent ENTER button push
void setup() {
enter=false;
lcd.begin(16, 2); //start LCD 16 x 2
pinMode(2, INPUT_PULLUP); //to be used for interrupt ENTER button
attachInterrupt (digitalPinToInterrupt(2), enterAscii, FALLING);
// set analog pin numbers for Arduino Mega
pinMode(A8, INPUT_PULLUP);// set pull-up on analog pin A8 for LSB of binary input mega pin #88
pinMode(A9, INPUT_PULLUP);// set pull-up on analog pin A9
pinMode(A10, INPUT_PULLUP);// set pull-up on analog pin A10
pinMode(A11, INPUT_PULLUP);// set pull-up on analog pin A11
pinMode(A12, INPUT_PULLUP);// set pull-up on analog pin A12
pinMode(A13, INPUT_PULLUP);// set pull-up on analog pin A13
pinMode(A14, INPUT_PULLUP);// set pull-up on analog pin A14
pinMode(A15, INPUT_PULLUP);// set pull-up on analog pin A15 for bit 8, MSB of binary input mega pin #82
pinMode(A7, INPUT_PULLUP);// on off test
}
void loop()
{
char ascii = 0x00;
int Switches = 0;
lcd.setCursor(0, 0); // set cursor position to start of first line on the LCD
lcd.print("My name is ");
// Turn off the blinking cursor:blinking cursor experiment
//lcd.noBlink();
//delay(3000);
// Turn on the blinking cursor:
//lcd.blink();
// Turn off the cursor:
// lcd.noCursor();
//delay(500);
// Turn on the cursor:rsor obn or off
// lcd.cursor();
// delay(500)
if (enter) //push button to post a new character
{
delay (200); // debounce
// lcd.print(n);//position of curser for troubleshooting
int Switches = readSwitches (Switches);
ascii = ascii + Switches; //ASCII character for switches value
lcd.setCursor(n, 1);// set cursor position to n of second line on the LCD
lcd.print(ascii);
n = n + 1;
enter=false;
}
delay (500);// wait after posting a new character
}
void enterAscii() //test of interrupt
{ static unsigned long last_interrupt_time = 0;
unsigned long interrupt_time = millis();
// If interrupts come faster than 300ms, assume it's a bounce and ignore
if (interrupt_time - last_interrupt_time > 300)
{
enter=true;
}
last_interrupt_time = interrupt_time;
}
int readSwitches ( int Switches ) // function to return value Switches
{
// Switches = 0;
if (digitalRead(A8) == HIGH)
Switches += 1;
if (digitalRead(A9) == HIGH)
Switches += 2;
if (digitalRead(A10) == HIGH)
Switches += 4;
if (digitalRead(A11) == HIGH)
Switches += 8;
if (digitalRead(A12) == HIGH)
Switches += 16;
if (digitalRead(A13) == HIGH)
Switches += 32;
if (digitalRead(A14) == HIGH)
Switches += 64;
if (digitalRead(A15) == HIGH)
Switches += 128;
return Switches;
}
Attachments
Step 5: Add Instructions
I tried to make the game self explanatory, with enough text to challenge and teach the concepts.
Here is a .pdf file of the text artwork I used for instructions as well as a table of ASCII equivalent binary codes and some additional trivia about bits, bytes, nibbles and hexadecimal. I would have provided a .doc file that you could edit, but Instructables doesn't support the format.
Attachments
Step 6: Enjoy!
I hope I have given enough information to allow you to build this instructive game. If you build it, I'd love to hear about your experiences.
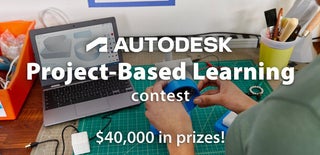
This is an entry in the
Project-Based Learning Contest
2 Comments
5 weeks ago
Very nice project!
Great idea for teaching as well!
Thanks for sharing,
Bob D
4 months ago
Thanks for sharing :)